With millions of Android
developers producing over a million apps annually, it is essential for these
mobile app developers to create apps that are feature-rich, compact, and
durable all at once.
However, there are countless
combinations and variants when it comes to hardware, software versions,
compatibility options, and other factors.
How do you ensure that your
apps are made for your broad audience, are quick, and provide you the ability
to just provide each user with the resources they require while still keeping
the app size as minimal as possible?
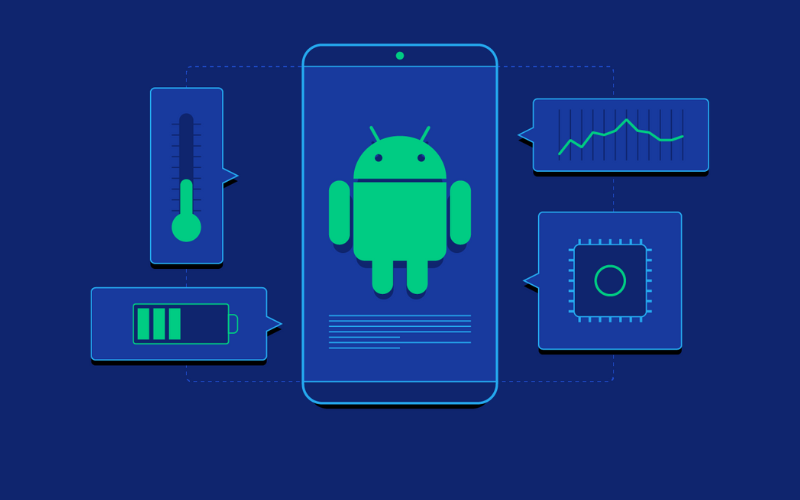
We’ve gathered some advice
and resources on how to improve Android app performance in this article.
1.Thread usage
optimization
There are two basic
categories of threads in Android that you must be aware of. The main thread,
also referred to as the UI thread, is in charge of handling user interaction
with the program and rendering the UI.
The second kind of threads
are background ones, which can handle prolonged tasks while the main thread
stays in place to control UI changes.
Your user interface will
always be refreshed every 16ms by the UI thread in order to maintain
satisfactory performance and a positive user experience.
Because of this, you should
never execute anything that requires a lot of time in the UI thread; otherwise,
you will go over the 16ms limit and your app will appear sluggish and
unresponsive.
Instead, you should always
run time-consuming operations in background threads.
2.Reduce the size of your app
Reducing the size of your
application is one of the simplest ways to optimize android app and enhance its
performance.
Users in emerging economies
generally avoid downloading programs that are too large, regardless of whether
their devices are connected to 26/3G networks or a pay-by-the-byte plan.
🔗 Use
Android App Bundles to publish your app
Posting your program as an
Android App Bundle, a new upload format is the simplest approach to obtain
quick app size savings when publishing to Google Play.
For each user's device, it
will produce APKs that are tailored to that device. It will only download the
materials and code needed for your app to run.
🔗 Utilize
the Android Size Analyzer
The tools mobile app
developers can use to improve Android app performance are numerous. The Android
Size Analyzer tool is one of them.
This tool makes it easier to
identify and apply a range of methods for reducing the size of your program.
It's accessible as an Android Studio plugin and a standalone JAR. Both as a
command line and as a plugin, you can utilize it.
3.Take into account low configuration devices
When developing apps, it's a
good idea to keep low-configuration devices in mind. It's improbable that every
Android user has more than 2GB of storage.
Smartphone performance can
be significantly impacted by memory-intensive apps. Apps are always being
optimized by developers for high configuration devices.
Consider a low-config
smartphones if you want to get the most out of your Android app's performance.
🔘 Related: Top Android App Development Fundamentals
4.Avoid deprecation
With each new version of
Android, certain APIs may become obsolete or need to be refactored to improve
the developer experience or enable new platform capabilities.
Android will occasionally
stop supporting outdated APIs and point developers toward new APIs.
🔗 Use
appropriate APIs
Use the correct APIs to
optimize android app. Using unneeded API might slow down Android's speed by
adding overhead to the system.
🔗 Refactor
the dependencies you have
Rearrange your dependencies
to suit your requirements and tastes. Utilizing the outdated API is unnecessary.
🔗 Avoid
abusing the system
Abusing the system will be
simpler if the deprecated APIs are used. It provides a point of access into the
system, making it vulnerable to abuse.
🔗 Keep
your tools and dependencies up to current at all times
You may improve Android app
performance by staying current with your dependencies and tools. The API will
be improved, improving performance.
5.Caching and Offline Mode
This is a method to use when
there is no network or when it is weak. It's always preferable to show something
than to show nothing at all.
To accomplish that, your app
should employ some form of caching, where it stores the data it receives from
the network (or another source) and displays it later.
This is particularly
effective if the majority of the data you load is the same each time the app is
launched.
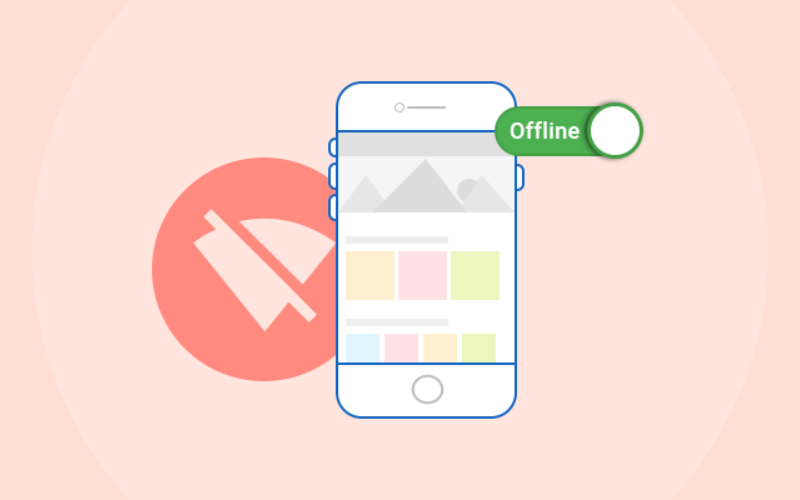
This enables you to lower
the server load, which in turn lowers the radio consumption on your mobile
device, improving battery life.
6.Optimize the Frame Rate
On iOS and Android, animations
and gestures can be rendered at a maximum frame rate of 60 frames per second
(FPS). Android performance will be sluggish if it is any slower.
To hit the 60fps goal, the
rendering code in the program must be executed in under 16 milliseconds.
If the garbage collection
time for your app is 5 milliseconds, the performance cost to the app can be
very high.
You get 58-59 frames per
second (fps) instead of 60 fps when your Android app misses the 16ms (60 fps)
limit once because it is unable to use the window for that frame. The app still
took 21ms to render, but since a window was missed, the user saw your app
screen as rendering twice as slowly.
7.Use modern picture formats
The most recent image
formats, such as WebP, which are smaller in size, adaptable, backwards
compatible, and optimized, can save a lot of space and resources when used for
static images that are converted from SVG for vector animations, etc.
8.Optimize memory usage
Random Access Memory (RAM)
is necessary to allow the user to multitask. Android sets a RAM restriction for
each program. These limitations are flexible and open to alteration.
Regular trash collection is
performed by both the Dalvik virtual machine and the Android Runtime (ART).
This does not, however, mean that you should ignore where and when your
software uses memory.
🔗 Eliminate
memory leaks
Memory leaks are a
phenomenon that happen when unnecessary space in memory is taken up by unneeded
things and the garbage collector is unable to gather and clear that space.
The issue here is that
references to these obsolete objects still exist. Let's imagine, for instance,
that we keep a reference to an inactive Activity in our code, which means that
we also keep all of the Activity's layouts, views, and other data.
Here are some suggestions for avoiding memory leaks and fixing
them:
- After your app has
finished utilizing an event or handler, always unregister it. Alternatively,
you can wait for the call to the on destroy method, which is the last call you
receive before your activity or component is destroyed.
- Keep Context Objects
out of your created classes. Some programmers frequently transfer the context
from activities to classes using Context, which is then stored in a private
variable.
-To discover the leaks
in your application, use LeakCanary or the Android profiler.
🔗 Reduce
the duration of services
The length of each service
must be kept to a minimum in order to improve Android app performance. If it
isn't correctly optimized, it could result in a resource deadlock. Controlling
the lifecycle of services is always a smart idea.
🔗 Release
UI resources when users change to another UI
When a user transitions to a
new UI, it's a good idea to release the resources that the old UI was using for
its onPause and onStop callbacks.
These resources frequently
take the form of broadcast receivers or database connections. By doing this,
there will be less strain on the resources, which will optimize android app UI
performance and result in an improved experience.
🔗 Use
memory-efficient programming
To minimize system overhead,
memory-efficient code should always be used.
Reduce
your reliance on outside libraries
With libraries, there is a
risk that the user will also want the dependencies. As a result, it is
challenging to remove dependencies from a packed aar-file.
Inconsistency may result if
only a portion of the library is packaged for your library but the user
requires a more recent version of the same library.
9.Refine the architecture
The most troublesome and
obvious defects are those that damage the lifecycle architecture.
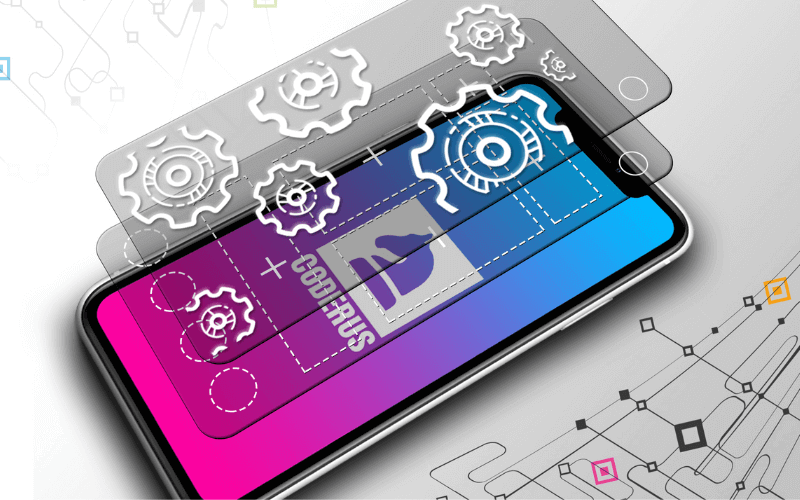
To prevent problems of this
nature, become familiar with the lifespan of app components, fragments, and
surrounding activities.
At all times, keep your
structure straightforward. The best course of action is to work with the
framework rather than against it.
🔗 A data source shouldn't be your app's entry
point
The most crucial rule to
remember is to refrain from writing all of your code in an Activity or a
Fragment.
These classes serve as the
interface between your program and the Android operating system. There should
only be logic for OS system interaction and Ul interface.
Because to user activities
or system issues like low memory, they may be removed at any time by the OS. To
provide a positive user experience and easier app maintenance, it's best to
lessen your reliance on them.
🔗 Set
up clear divisions between the app's various parts
It's crucial to establish
distinct boundaries between the various parts of an Android application. It
will be useful if mobile app developers need to add, remove, or test
adjustments to the other modules of the program.
10.Balance the use of text and animations
Avoid overdoing it with your
app's animations or one particular screen. This may not be appropriate for a
fluid user flow and distracts users.
Where necessary, strike a
balance between text, animation, and hints. Users must be able to perform
certain activities simply looking at the animations.
🔘 Related: The Most Important iOS Development Tips
11.Establish a design system
Determining the visual
hierarchy for the entire app is always a recommended idea. If every other
screen has a distinct animation or transition, users will find it annoying.
The transitions should
ideally remain consistent for the same components across the whole program;
that is, all activity transitions should offer the same transition experience.
12.Optimize launch time
When using your application,
one of the first things consumers will notice is how long it takes to launch.
To prevent user annoyance, your software must launch and begin as rapidly as
feasible.
There are some guidelines to
abide by that will hasten launch time:
âž• On the user's first screen, don't instantiate too many views
âž• Don't bloat the UI with features you don't need when it first
launches
âž• Avoid using costly content decoding, such as bitmaps
âž• To track and
accelerate the launch of your app, use Android Vitals or Firebase Performance
Monitoring
âž• Keep your application
object's initialization light.
Conclusion
Any mobile app's performance
is vital; if it's sluggish and unresponsive, users will probably disregard it.
Likewise, the better your software performs, the more probable it is to be a
commercial success.
Mobile app developer need to
focus more on this and spend more time working to improve Android app
performance.
If you are looking for ways
to optimize android app you have created, feel free to contact us.